In this article, we'll break down the Stripe Checkout Session, a powerful tool for developers looking to integrate payment processing into their applications. Whether you're just starting with Stripe or looking to refine your implementation, this guide will cover everything you need to know. We'll discuss the basics of setting up your Stripe account, creating checkout sessions, handling payment outcomes, and customizing the checkout experience. By the end, you should have a solid understanding of how to effectively use Stripe Checkout Sessions in your projects.
Key Takeaways
- Stripe Checkout Sessions simplify payment integration for developers.
- Setting up your Stripe account is the first step to using its features.
- Creating a checkout session involves defining API routes and redirecting users.
- Handling payment success and failure is crucial for user experience.
- Customizing the checkout experience can lead to better conversion rates.
Getting Started With Stripe Checkout Session
Understanding the Basics
When you first step into using Stripe Checkout Session, you’re stepping into a much simpler way to handle payments. Instead of building everything from scratch, you can rely on Stripe to take care of card processing details. You set up a session that bundles payment method types, order items, and redirection URLs. For example, here’s a quick look at the main parameters:
Getting started is easier than it sounds. Try to imagine you are setting up a small shop that uses a ready-made checkout solution like Stripe checkout page to handle orders effectively.
Setting Up Your Stripe Account
Before you can integrate anything, you need an active Stripe account. The process is pretty straightforward if you follow these steps:
- Create your account on Stripe’s sign-up page.
- Verify your email and complete the onboarding setup.
- Retrieve your API keys from the dashboard so you can connect your code to Stripe.
Once your account is up, you’re ready to progress to development and configuration, ensuring your account’s settings match what your application needs.
Integrating Stripe in Your Application
Integrating Stripe into your application might sound like a daunting task, but it’s much more approachable once you break it down:
- Install the Stripe package and any dependencies in your project.
- Create an API route where you call Stripe’s session creation method.
- Set up your front-end to trigger the session creation and handle redirection to the checkout interface.
Remember, testing your integration in a sandbox environment is key before you go live.
Setting up Stripe in your application might initially seem challenging, but with each step you take, you find that it becomes more manageable. This process ensures that you can handle payments reliably and safely in your own app.
Creating a Checkout Session
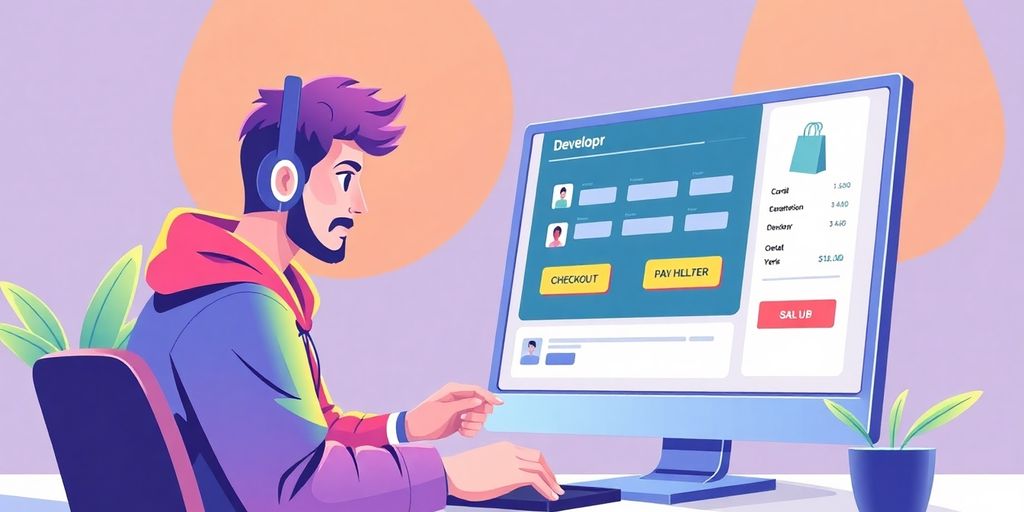
Defining Your API Route
When you start building your checkout session, you first need a dedicated API route to handle session creation. Generally, you'll set up an endpoint that listens for POST requests. This endpoint—often something like /api/checkout-sessions/create
—serves as the gateway for all checkout-related calls.
You should:
- Configure your server to accept POST requests.
- Validate incoming data to avoid issues later on.
- Handle potential errors early in the process.
Tip: Always test your API route separately before integrating it into your main application flow.
Building the Checkout Session
This is the heart of your checkout process. Once your API endpoint is set up, you’ll use Stripe’s functions to create a session. Typically, you’ll call a function like stripe.checkout.sessions.create()
that requires details including payment method, line items (like product name, price, and quantity), and URLs for success and cancellation scenarios.
Steps you usually follow:
- Initialize your Stripe client with your secret key.
- Define the payment methods and add your line item details.
- Set up the success and cancel URLs, sometimes using placeholders like
{CHECKOUT_SESSION_ID}
to insert the active session info.
A quick reference table could look like this:
Don't forget to check out the useCheckout hook to get session data and handy functions while you build. This step solidifies the base upon which your checkout experience stands.
Redirecting Users to Checkout
After building your session, it’s time to redirect users. Once the session is ready, Stripe returns a URL where users can finalize their payment. The redirection process should be smooth and clear:
- First, retrieve the session ID from your API response.
- Then, use that session ID to redirect the user to the payment page.
- Finally, manage any cancellations or failures gracefully, providing clear feedback.
Here’s a quick checklist:
- Receive and verify the session details.
- Redirect users immediately using the URL from Stripe.
- Display appropriate messages in cases of success or cancellation.
Remember: A fast and clear redirection keeps the experience friendly and trustworthy.
Handling Payment Success and Failure
When you're using Stripe Checkout, you need to be ready for both wins and losses. Sometimes, a payment goes through without a hitch, and other times, things don't work out. In this section, you'll learn how to deal with the different outcomes gracefully. Remember, if your process involves various payment methods then it’s good to be prepared for any twist in the transaction tale.
Redirecting After Payment
After a user completes the payment process, you'll want to send them to the right page. For instance, if the payment gets accepted, they should land on a thank you or order summary page. If things go wrong, they might need a friendly error page that guides them on what to do next.
Here's a quick list on how to structure your redirection:
- Check the payment status once Stripe responds.
- Redirect users to a success page if the payment goes through.
- Send users back to a cart or a retry page if payment fails.
If you want a visual snapshot, here’s a simple table comparing the two scenarios:
Displaying Success Messages
Once a payment succeeds, it's time to celebrate a smooth transaction. Your success page should clearly display important details like the order summary, date, and a confirmation number for future reference. You should make success feel like a win by reinforcing each step with a neat, concise message.
Consider listing these details in a bulleted format:
- Order number and details
- Date of transaction
- Amount paid
- Any additional instructions for tracking your order
This approach not only confirms the successful payment but also builds the trust that things are handled properly.
It’s good to remember that clarity on the success page helps users know that everything is in order, especially if they end up wondering where to find their order details later on.
Managing Failed Transactions
Not every payment will go off without a hitch, and it's important to smoothly handle failures when they occur. In many cases, enabling a retry or providing clear support options can prevent frustration.
Here are some key steps you can take when a transaction fails:
- Inform the user that the payment didn't go through.
- Offer clear instructions on what to do next, whether it's trying again or contacting support.
- Consider even using a countdown or notification if the payment is under review.
By following these steps, you minimize user confusion and promote a better overall checkout experience. Also, remember that a quick redirect to a friendly, helpful page can ease user anxiety and improve their overall payout journey.
Customizing the Checkout Experience
Styling the Checkout Page
When you want your checkout page to feel like a natural extension of your site, styling is the way to go. You can adjust colors, fonts, and layouts so the page matches your overall look, keeping things neat and friendly. For tips on setting up a matching design, check out our custom checkout design.
Here are some quick pointers to get you started:
- Pick a color scheme that reflects your brand's vibe.
- Use simple, readable fonts.
- Keep the layout uncluttered so users focus on completing their purchases.
Getting your style right is half the battle.
Adding Upsell Opportunities
Upselling at checkout can be done subtly without feeling pushy. You want to offer smart suggestions that complement the user's choice rather than overwhelm them. A nudge here and there can increase your average order value. For more on this, refer to our upsell integrations.
Consider these strategies for effective upselling:
- Introduce related add-ons that enhance the main purchase.
- Highlight bundle offers or discounts for multiple items.
- Offer premium services or warranty extensions at a slightly higher price.
Configuring Payment Methods
A smooth checkout experience often depends on letting users choose from a range of payment options. By offering several payment methods, you cater to a wider audience and make the process smoother all around. Dive into our payment options for a deeper look at what you can offer.
Below is a brief look at possible payment methods you might configure:
This structured approach will help you cater to many users and reduce friction during checkout.
Best Practices for Stripe Checkout
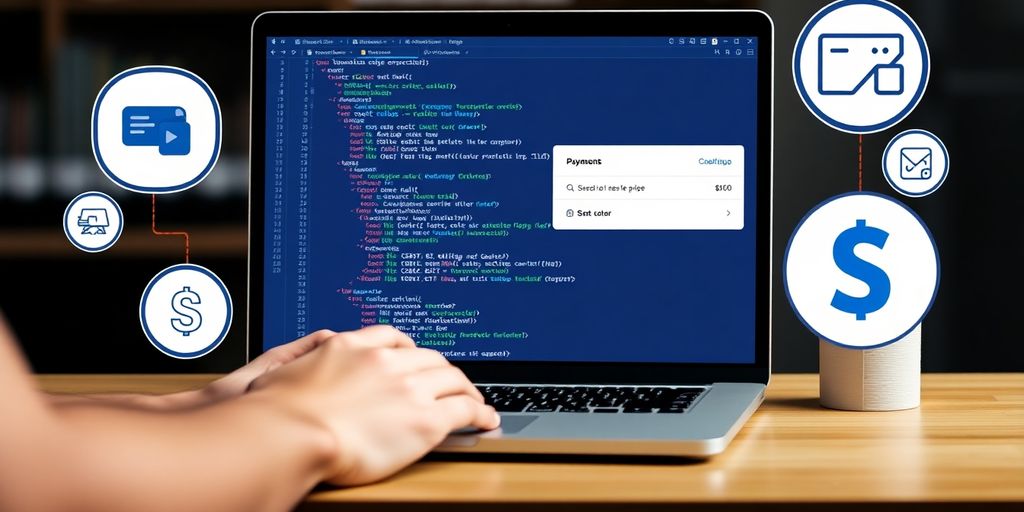
Optimizing User Experience
When you set up your checkout page, you want your customer to feel like you're speaking directly to them. Start by keeping forms simple and direct. Here are a few ways to keep things clear:
- Use clear labels for every field
- Keep the number of steps to a minimum
- Make error messages plain and helpful
Make your checkout process smooth to keep customers happy. Also, if you need inspiration, check out billing design for a few neat ideas.
Sometimes a few tweaks in the layout can make the experience so much friendlier for your users.
Ensuring Security
Security in your checkout is just as important as easy navigation. When you're handling money and personal info, you need to be careful. Consider these steps:
- Use updated encryption methods
- Regularly audit your code and dependencies
- Display trust signals to reassure your customers
Taking the time to check these details can really set your checkout apart. Also, think about reviewing billing design to see how good layouts handle security signals.
Monitoring Transactions
Keeping an eye on what happens during every checkout gives you better control over your business. Track performance by monitoring key points. You can even set up a basic table to watch your numbers:
Review the numbers from billing design for extra insights on maintaining a tight checkout process. By keeping these figures in check, you can adjust quickly when things seem off.
Troubleshooting Common Issues
Debugging Checkout Sessions
When your checkout session isn’t behaving as expected, start by combing through your logs. It might sound simple, but every little error can give you a clue. Clear logs are key to pinpointing the issue. You’re going to want to check these things:
- Document any error messages or unusual responses.
- Compare your API calls with what Stripe expects.
- Verify that your configuration hasn’t been accidentally altered.
Sometimes, it helps to organize info in a table. For instance:
Keep in mind that payment methods misconfigurations can also be a culprit when debugging sessions.
Resolving Payment Failures
If payments are failing, it’s time to get methodical. Step back and verify all the basics:
- Check that the customer’s card details are up to date, including expiration dates.
- Bring your attention to your API keys—make sure they’re the right ones and still active.
- Use test data with a known working sandbox card to isolate the issue.
- Make a note of any specific error codes, then consult Stripe’s guidelines on those codes.
These steps will help you rule out typical glitches and pinpoint the real problem, making it easier to fix things.
Contacting Stripe Support
If after all these tests your checkout is still acting up, it might be time to reach out to the Stripe support team. When you do this, keep your notes handy:
- Write down and include every error message you’ve encountered.
- Itemize all the troubleshooting steps you’ve already taken.
- Have your transaction IDs and checkout session details ready.
Having a well-organized summary can really smooth out the process when you need expert help. It cuts down on back-and-forth and speeds up getting your issue resolved.
If you're facing problems, don't worry! Many people run into common issues, and we have solutions for you. Check out our website for helpful tips and guides to get you back on track. Don't let these bumps slow you down; visit us today!
Wrapping It Up
So there you have it! We’ve walked through the ins and outs of setting up a Stripe Checkout session in your Next.js app. It might seem a bit overwhelming at first, but once you get the hang of it, it’s really not that bad. Just remember to keep your API keys safe and refer back to the Stripe docs whenever you need a hand. Whether you’re selling a single product or managing a full shopping cart, Stripe has got your back. Now, go ahead and make those payments happen smoothly. Happy coding!
Frequently Asked Questions
What is Stripe Checkout?
Stripe Checkout is a tool that helps businesses accept payments online easily and securely.
How do I set up a Stripe account?
To set up a Stripe account, go to the Stripe website and sign up by providing your email and creating a password.
What is a Checkout Session?
A Checkout Session is a process where a customer can pay for items in a secure environment provided by Stripe.
How do I create a Checkout Session?
You can create a Checkout Session by making a request to Stripe's API and providing details like the items being purchased.
What happens after a payment is made?
After a payment is successful, customers are redirected to a success page, and you can show them a confirmation message.
How can I troubleshoot payment issues?
If there are payment issues, check the error messages from Stripe and ensure that your API keys are correct.